Getting started
Meet Wisepops: your onsite marketing platform
Getting started with popups
Getting started with notifications
Onsite marketing performance benchmark and best practices
Navigating Wisepops Dashboard
App & features
Popups, sticky bars and embeds
Popups
How to create a popup for mobile
How to create a Popup: A Step-By-Step Guide
How to add a tab to your popup campaigns
Bars
Embeds
Campaign builder
Design
Create a multi-step campaign
Use a custom font in your campaign
Closing Options
Adding a Countdown Timer
Write Your Own CSS Rules into Your Popups
JS Callbacks
Introduction to JS Callbacks
After-popup-close Event
After-tracked-click Event
After-form-submit Event
Before-form-submit Event
Before-popup-display Event
Before-tab-display Event
Before-tracked-click Event
Scheduling your popup campaigns
Navigating the Popup Campaign Builder
A/B Testing
Campaign Management
Publish or Pause Campaigns
How to duplicate (and move a campaign)
Archiving, Deleting or Restoring campaigns
Integrations
ESP Integrations
Connect Wisepops with Splio
Connect Wisepops with Mad Mimi
Connect Wisepops with Campaign Monitor
Connect Wisepops with Emarsys
Connect Wisepops with Ometria
Connect Wisepops with Experian (Cheetah)
Connect Wisepops with Optimizely Data Platform (ex Zaius)
Connect Wisepops with Selligent
Connect Wisepops with Actito
Connect Wisepops with Acoustic
Connect Wisepops with Voyado
Connect Wisepops with Listrak
Connect Wisepops with Omnisend
Connect Wisepops with Constant Contact
Connect Wisepops with GetResponse
Connect Wisepops with SmartrMail
Connect Wisepops with Keap (formerly Infusionsoft)
Connect Wisepops with Drip
Connect Wisepops with MailChimp
Connect Wisepops with ActiveCampaign
Connect Wisepops with Brevo (ex Sendinblue)
Connect Wisepops with Salesforce
Connect with Mailjet
Connect Wisepops with ConvertKit (through Zapier)
Sync your leads with Shopify
Connect Wisepops with HubSpot
Connect Wisepops with SendGrid
Integrating Wisepops with Klaviyo
Connect Wisepops with dotdigital
Connect Wisepops with Iterable
Connect Wisepops with Customer.io
Connect Wisepops with Intercom
Connect Wisepops with Pipedrive
Integrate Wisepops with your ESP using Zapier
Connect Wisepops with AWeber (through Zapier)
Connect with Close.io (through Zapier)
SMS Integrations
Connect Wisepops with Emotive
Connect Wisepops with Postscript
Integrate Wisepops with Attentive Using Zapier
Other integrations
Zapier
Send your leads to a Google Spreadsheet (using Zapier)
How can I take advantage of the Zapier integration?
Send a notification when an email is submitted (through Zapier)
API docs
Connect Wisepops with Yotpo SMS and Email
Notifications
Notification builder
Integrations
Mastering Notifications: boost customer journeys with Wisepops
How to send push notifications
How to prioritize and pin a notification at the top of your Notification Feed
Display rules
Triggers
Target a Popup to Display on link or CTA Click
On scroll trigger
Target a Popup To Display on Hover
Adding a delay to your popup
Page targeting
Audience
Targeting new vs. returning visitors
Chrome’s Update and Its Impact on Source URL Targeting
Target by traffic channel, source or UTM parameters
Target a Specific Campaign
Target or Exclude a Specific Country, Region or City
Targeting campaigns to a specific language
Frequency
Advanced targeting
Set Custom Events to Trigger Popups
Using Custom Properties and Events for contextual targeting in Wisepops
Set up Custom Properties for Wisepops and use them to target your campaigns
How To Test Your Custom Properties
Shopify Built-In Properties
Use Google Tag Manager Variables as Custom Properties
Use Shopify Liquid Variables as Custom Properties
Set Custom Properties Based on Cookie Values
Default Custom Properties in Magento
How to target Klaviyo Segments in Wisepops
Use cases
Upsell & cross sell
Gamification
Create a Spin-the-Wheel Popup Without Collecting the User’s Email Address
Create a Spin-to-Win Campaign
Guidelines for writing game rules
List growth
Create a Thank You Message (or Display a Coupon) After Sign-Up
Leverage 2-Tap SMS with Wisepops
Collect the URL on which the popup form is submitted
How to handle required merge fields
Add Custom Validation to Your Signup Forms
Add a product to the cart when a CTA is clicked (Shopify)
Collect the UTM parameters when a popup form is submitted
Adding more fields to your form
Select the dates available in your forms
Collect phone numbers / SMS opt-ins with Wisepops
How to pass Dynamic Variables into Hidden Fields
Send the data collected by a form to the redirected URL
How to grow your email list using the notification feed
Geo Targeting
Surveys
How to create a survey with Wisepops
Embed a SurveyMonkey Quiz Into a Popup
Display a form field conditionally
How to create an NPS survey popup
Embed a Typeform in Your Popup
Video
Promote discounts
How to create unique Shopify discount codes in your popups and notifications
Apply a Shopify discount code to the cart
How to use discount codes in Wisepops
Referral
Cart or site abandonment
How to create a cart recovery popup with Wisepops
How to create a cart recovery popup with Wisepops (Magento)
How to create a cart recovery popup with Wisepops (Shopify)
Creating a Post-Purchase Survey
Create an Exit Popup
How to create a lead magnet with Wisepops
Advanced use cases
Trigger a popup from the notification feed
Dynamically Add a Custom Parameter to a Redirect URL
Fire the Facebook/Meta Pixel When a form is Submitted
Forbid Disposable or Free Emails in Your Signup Form
Display a Popup Only After Another One Has Been Seen (or Clicked)
Personalize the Content of Your Popup or Notification on the Fly
Apply filters to your dynamic variables
Inject the Name of the Visitor's Company into Your Popups (with Clearbit)
Display the visitor's city within your popup
How to Target (or Exclude) Visitors Who Have Seen a Specific URL
How to retarget visitors who saw a specific page on your website
Display a Popup Based on an Alexa Rank Condition (With Clearbit)
Insert social widgets into your campaigns
Flag a Popup as Clicked With JS Code
Display a campaign when a product is added to the cart (Shopify)
Close a Popup With JS Code
Stop showing a notification after clicking on a popup
Analytics & Reporting
Goal Tracking
How to setup Goal Tracking for Wisepops
How to Set Up Goal Tracking in Magento
How to set up Goal Tracking using Google Tag Manager
Google Analytics
Introduction to the Google Analytics integration
Send Wisepops events to GA4 using GTM
Track clicks on your links using Google Analytics
How to use the Wisepops events in Google Analytics
Disable Wisepops on my device
How to Filter Out Internal Traffic
How to track clicks in custom HTML blocks
Time zone used for reporting
Exporting your data
Analytics: Understanding how your campaigns perform
How to track signups with a tracking pixel
Exclude your device
Account & Setup
Install the Wisepops tracking code
CMS integrations
Installing Wisepops on your website via Google Tag Manager (GTM)
Add Wisepops to Zoey
Add Wisepops to Webflow
Add Wisepops to Instapage
Add Wisepops to Blogger
Check your code implementation using Mozilla Firefox
Add Wisepops to Big Cartel
Add Wisepops to Drupal 8
Add Wisepops to BigCommerce
Add Wisepops to Umbraco
Add Wisepops to Joomla
Add Wisepops to Squarespace
Add Wisepops to Magento 2
Add Wisepops to Google Sites
Add Wisepops to Cratejoy
Add Wisepops to 3dcart
Add Wisepops to Prestashop
Add Wisepops to Shopify
Add Wisepops to Weebly
Add Wisepops to Segment.com
How to add Wisepops to a custom website?
Add Wisepops to Wordpress/WooCommerce
How to add Wisepops to a Wix website
Add Wisepops to Volusion
Add Wisepops to SiteBuilder
Add Wisepops to Drupal 7
Add Wisepops to Neto
Wisepops on Single-Page Applications
Add Wisepops to Mono
Add Wisepops to Opencart
Integrating Wisepops with your website
Check your code implementation using Google Chrome
Adding the notification feed to your website
Custom Setup Guides for Shopify Themes
Minimal Theme
Dawn theme
Narrative Theme
Debut Theme
Simple Theme
Express Theme
Venture Theme
Brooklyn Theme
Boundless Theme
Supply Theme
Adding the notification feed to your website
Customize your Notifications Feed with Advanced Feed Customization
Add the notification feed with Shopify
Hide the bell until the notifications are loaded
Billing
🔗 Integrations with your tools
Add/Delete Website
Add, edit or delete users and manage permissions
Use Distinct Websites for Staging and Production
Enabling Right-to-Left Language Support
Uninstall Wisepops from your Shopify website
How to I remove the notification feed from my site
Can I use Wisepops on multiple domains?
Security & Compliance
Legal
GDPR & CCPA compliance
Configure the Wisepops cookies lifetime
Accessibility and compliance with ADA/WCAG
Information concerning cookies
Compliance with Shopify's Customer Privacy
Security
FAQ
Preview/test your campaign on a hidden URL
Popup Design FAQ
Will Wisepops slow down my website?
Exclude Your Existing Subscribers
Is Wisepops compatible with my mobile app?
Can I show more than one popup on the same page?
Troubleshooting
How to fix email synchronization issues
Troubleshooting: Why isn't my campaign appearing?
Tips for effectively reporting an issue
Troubleshooting: Dealing with Ad Blockers and VPNs
Collected emails don't appear on my dashboard
Why does my popup look broken?
My emails are not collected in Mailchimp
My Popup is Locked!
Why does my popup appear for a few seconds and disappear?
- All Categories
- App & features
- Use cases
- Surveys
- Display a form field conditionally
Display a form field conditionally
Updated
by Boris Hocdé
You can make a form field appear only if another field matches a condition. For example, you can your visitors how they heard about you. In a dropdown, the visitor can choose the 5 most frequent channels. And a 6th option "Other" makes a free text field appears.
First, create 2 form fields
The first one should be a dropdown to select the default choices:
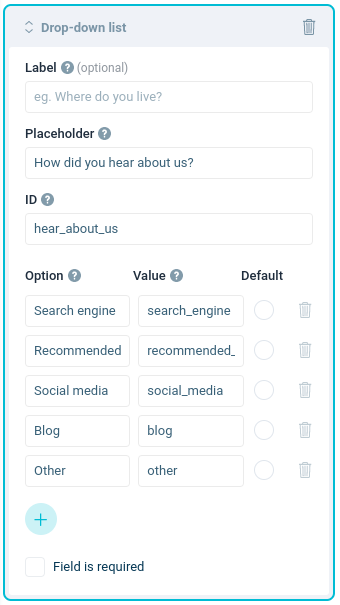
And the second one is for the free text in case "Other" is selected.
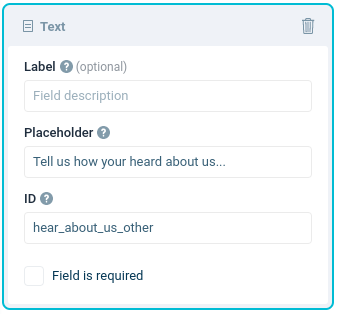
The IDs of the two fields and the value of the "Other" option are used to connect things together.
In our example, we use the field IDs hear_about_us
and hear_about_us_other
, and the special value other
.
In CSS, hide the free text field unless it has a class to make to visible
In our custom CSS tool, add the following snippet to make the free text field appear only if it has a class visible
:
[name=hear_about_us_other]:not(.visible) {
display: none;
}
Pay attention to replace hear_about_us_other
by the field ID you chose in your form.
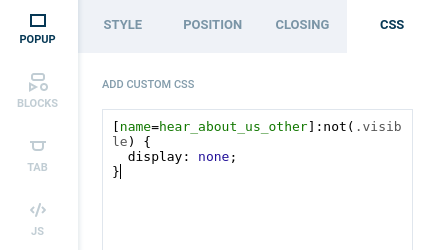
In JavaScript, add the visible class when relevant
Now, let's add and remove the class visible
to the free text field when it should appear or disappear.
Create a JS callback listening for the event Before popup display, with the following snippet:
var customIfValue = 'other';
var choiceField = event.target.querySelector('select[name=hear_about_us]');
var customField = event.target.querySelector('input[name=hear_about_us_other]');
if (choiceField && customField) {
choiceField.addEventListener('change', function() {
if (this.value === customIfValue) {
customField.classList.add('visible');
} else {
customField.classList.remove('visible');
}
});
}
Ensure to replace other
, hear_about_us
, and hear_about_us_other
by the values you're using in your form.
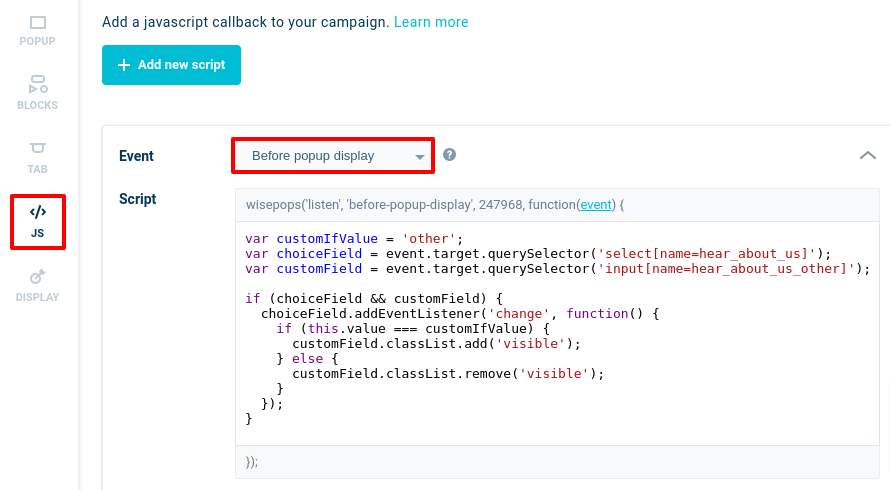
And voilà! Your free text field will appear if "Other" is chosen. And disappear if another option is selected.
To go further
If you want to use a checkbox or multiple choice field for condition
The script differs slightly (choiceField and second "if" condition):
var customIfValue = 'other';
var choiceField = event.target.querySelector(`input[type=checkbox][value=${customIfValue}]`);
var customField = event.target.querySelector('input[name=hear_about_us_other]');
if (choiceField && customField) {
choiceField.addEventListener('change', function() {
if (this.checked) {
customField.classList.add('visible');
} else {
customField.classList.remove('visible');
}
});
}
If you want to use a radio field for condition
The script differs slightly (choiceField and second "if" condition):
var customIfValue = 'other';
var choiceFields = event.target.querySelectorAll(`input[type=radio]`);
var customField = event.target.querySelector('input[name=hear_about_us_other]');
if (choiceFields && customField) {
choiceFields.forEach((option) => {
option.addEventListener('change', function() {
if (this.checked && this.value === customIfValue) {
customField.classList.add('visible');
} else {
customField.classList.remove('visible');
}
})
});
}
If you want to have 2 conditional fields for the same dropdown
This script adds other value to check if it should be revealed. It removes visible tags for all fields and adds visible for the condition that's met.
If you wanted to create other condition, add hidden field analogically as the first (add field, hide it with CSS) and add slightly modified script. The code below assumes the 2nd input has name `another_field_to_reveal` and value of select option is `another`. These are just example names and can be named freely:
var choiceField = event.target.querySelector('select[name=hear_about_us]');
var customIfValue = 'other';
var customField = event.target.querySelector('input[name=hear_about_us_other]');
var customIfValue2 = 'another';
var customField2 = event.target.querySelector('input[name=another_field_to_reveal]');
if (choiceField && (customField || customField2)) {
choiceField.addEventListener('change', function() {
customField.classList.remove('visible');
customField2.classList.remove('visible');
if (this.value === customIfValue) {
customField.classList.add('visible');
} else if(this.value === customIfValue2) {
customField2.classList.add('visible');
}
});
}
If you want to use dropdown as a field to reveal
There are slight CSS and JS differences.
CSS:
.wisepops-field[name=hear_about_us_other]:not(.visible) {
display: none;
}
JS - customField line is different:
var customField = event.target.querySelector('.wisepops-field[name=hear_about_us_other]');
If you want to remove space created by hidden field:
Hidden fields still add some margins (set in "Size" menu -> "Spacing" available in top toolbar after selecting the form block). If you want to get rid of extra space when field is hidden:
1. Reduce the Spacing to 0
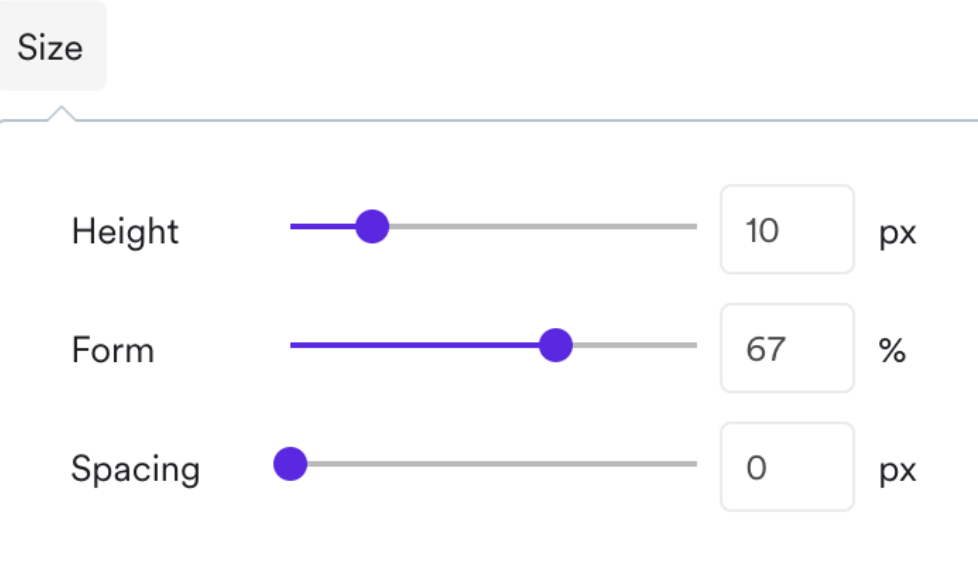
2. Add CSS to apply spacing to `.wisepops-field` container (1 level below editor's spacing is added):
div.wisepops-field, button {
margin-top: 12px;
}